From Bricks to Views: Hierarchical Architecture 101 ๐งฑ๐จโ๐ซ
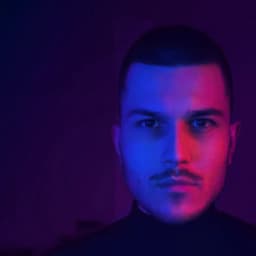
ลukasz Fiuk
Why Code Structure Matters? ๐ค
In the world of agile web development, code structure is the hidden hero of efficiency, maintainability, and scalability. But why architecture is it so important? Let's see:
๐ฃ Readability: When code is well-structured, it's easier to read and understand. This is not just beneficial for the original developer, but crucial for any one who needs to review, debug, or build upon that code.
๐ Reusability: A good code structure promotes reusability. When we structure our code into self-contained, modular components, we can use these components in multiple places throughout our application (and beyond!).
๐ง Maintainability: You'll have to modify, fix and maintain your code. This might become a painful experience if your codebase is messy. Organized structure reduces the chance of creating bugs in seemingly unrelated parts of the application.
๐ Scalability: Clean code is easier to scale. Consistent methodology and conventions make your code predictable and therefore easy to build upon.
๐ง๐ปโ๐คโ๐ง๐พ Collaboration: Finally a sound code structure simplifies collaboration. It's much easier to work your teammate code, if you are all working with the same patterns.
I believe that many projects suffer from a weak file structure because it's easy to overlook or ignore this aspect during the initial stages of development. However, as the project progresses, reconfiguring the structure becomes a hell of a task that nobody has the time for. โ ๏ธ
How to do it right? Quick look at Hierarchical Architecture ๐ค
Here's a little sneak peek into how I like to structure my code: ๐๐ช
๐พ >_ txt/src/ โโโ 1_components/ โโโ 2_sections/ โโโ 3_containers/ โโโ hooks/ โโโ states/ โโโ styles/ โโโ types/ โโโ utils/ โโโ anything else that you need
As you may have noticed, the first three folders are numbered. This intentional strategy aims to visually order the most critical project folders and impose a hierarchy. ๐ช
The core idea revolves around partitioning the project into three main blocks. Imagine constructing a house: ๐
1_components
: Components are our foundational building blocks. They should be simple, reusable, and generic. In the context of web development, a component is a reusable, self-contained piece of code that encapsulates a piece of functionality or user interface. Examples might include aButton
or aTypography
component.2_sections
: Sections are like the walls of our house. Each section represents a specific fragment of the page and is composed of multiple components. They are more specific than components and often contain styles and positioning unique to that section. Examples may include aNavbar
,Footer
, orHeroBanner
.3_containers
: Containers are the rooms in our house. Each container is composed of several sections and represents a particular view, such as aHomePage
,ProductPage
orProfilePage
.
This approach is dead simple, readable and allows for great flexibility both for developers, and clients. In fact, it is a simplified blend of Atomic Design, and BEM methodologies - preserving their principles while emphasizing simplicity.
Rules and Definitions ๐๏ธ
Components ๐งฑ
Components are the basic building blocks of the application. They are self-contained, reusable pieces of code that encapsulate specific functionality or user interface elements.
- No Positioning ๐บ: Components shouldn't be positioned. Their placement should be determined by the parent section in which they're used.
- Simplicity and Encapsulation ๐: Components should be simple and encapsulated. All things related to a single component should live within the same folder.
- Generality ๐ง: Components should be generic, designed to be used in multiple contexts throughout the application.
- Tested ๐งช: If possible, components should be tested as they will be reused in multiple places. This will help to ensure their robustness and reliability.
- Extra Care โค๏ธ: Components should be written/edited with extra care, as they are meant to be reused in many places, possibly across many projects.
Component Folder Structure Example: ๐๏ธ
๐พ >_ txtButton/ โโโ Button.tsx โโโ Button.styled.ts โโโ Button.utils.ts
You can group components as you see fit. For example:
๐พ >_ txtButtons/ โโโ PrimaryButton/ โโโ SecondaryButton/
Sections
Sections are larger blocks of content made up of various components arranged together.
- Subfolders for Complexity ๐๏ธ: If a section is complex, create subfolders to split code into smaller pieces.
- Composition ๐งฉ: Aim to use composition to make these blocks more versatile.
- Reuse Components ๐ฐ: Sections should be built from reusable components, and elements specific to the section, such as wrappers etc.
- Uniqueness ๐: While components are generic, sections should have a specific purpose in the application.
Containers
Containers are specific views or templates within an application. They are constructed from one or more sections and if needed - wrappers.
- Built from Sections๐งฉ: Containers should be primarily built from sections, not single components. This keeps the complexity of each part manageable and enforces the hierarchy of components -> sections -> containers.
- Single Responsibility ๐: Each container should have a single responsibility. This follows the single-responsibility principle (SRP), making the code easier to maintain and understand.
- Naming Convention ๐ฟ: Containers should be named based on their functionality. For example, a container for the homepage could be named
LandingPage
and template for blogpost could be namedArticlePage
Component Driven Development ๐ฆ
Building large applications or websites isn't easy because usually there are many abstract connections and concepts that you have to constantly keep in mind. With tangled pieces and large, unfocused components, things could quickly get out of hand.
Because of this, it's a good idea to focus on individual parts of your app at a time. Take your time to build generic components, and consider creating a UI library with tools such as Storybook to work in isolation. ๐ Add tests and check if your components are up to spec. If you are using Storybook you might want to check Chromatic for automated UI tests. ๐งช
Once the generic components are in place, building larger parts will be much simpler, and you'll avoid a lot of frustration ( trust me! ). While I'm aware that it's not always possible, as designs may evolve as you go, I firmly believe that striving to work in this manner is the way to go. โจ
Final notes ๐ธ
I think that it is important to acknowledge that there is no 'one-size-fits-all' solution. Just as every digital project is unique, with its own set of goals, challenges, and scope, the structure that best supports it will vary.
While there may be no single 'perfect' structure, the importance of adopting some form of organized structure is undeniable.
I truly believe that upgrading 'small' things such as code structure or file naming conventions had one of the biggest positive impact on my coding in general. Nice, clean and consistent code brings peace and joy to my heart and I hope you'll like it too. Happy Hecking! ๐